Tips_tutorials   >   Studio101   >   Window Class
Window Class
In this section we will create a window class which will list all of the country records in a complex grid where users will be able to add and change country records. We will use the Omnis Studio smartlist feature to take care of tracking any changes to the list and to save changes to the database.
Create a Window Class
To create the window class:
- F2 Browser > select Contacts library > click New Class > click Window.
- Name the window class, wCountryList.
- F6 Property Manager > General tab > set the $title property to Countries.
- Select wCountryList in the F2 Browser.
- Press F8 to go to the window class methods.
- Select the $construct method and enter the following code:
Do iList.$definefromsqlclass('tCountry')
Do iList.$sessionobject.$assign(dbsessionobj)
Do iList.$getAllRecords() Returns FlagOK
If FlagOK
Do iList.$smartlist.$assign(kTrue)
End If
Quit method FlagOK
The $construct window class method code will run when the window is instantiated, just prior to it becoming visible in the display.
- Press F3 to open to the window class editor window.
Add a Complex Grid
We will now add a complex grid field object to the wCountryList window class.
- Press F3 to open the Component Store.
- Drag the Complex Grid from the component store to the wCountryList window class.
- With the complex grid component selected in the window class, press F6 to open the Property Mananger.
- Set the following properties in the complex grid:
General tab
$name - CountryList
$dataname - iList
$left - 10
$top - 10
$height - 200
$width - 150
Appearance tab
$columns - 2
$horzscroll - kFalse
$showhorzheader - kFalse
$showvertheader - kFalse
$vertscroll - kTrue
Action tab
$enterable - kTrue
$extendable - kTrue
- Double-click the CountryList complex grid object in the window class to get to the object's methods.
- Right-click CountryList in the treelist > select Insert New Method.
- Name the new method $construct. This field object $constructmethod will run prior to the window class $construct method.
- Enter the following code in the CountryList complex grid's $construct method.
Do $cfield.$edgefloat.$assign(kEFposnClient)
This sets the complex grid object to expand to fill the area of the window class when the window is instantiated. By setting kEFPosnClient in the field object's $construct method you avoid having the component covering the entire window while you are designing the window class.
- Close the method editor to return to the window class editor.
- Drag an Entry field object from the F3 Component Store into column one of the complex grid.
- With the entry field object selected in the complex grid, set the following entry field properties in the F6 Property Manager:
General tab
$name - CountryName
$dataname - iList.CountryName
Appearance tab
$edgefloat - kEFposnClient
$horzscroll - kTrue
- Click the Background Objects icon in the F3 Component Store toolbar.
- Drag a Label field object from the F3 Component Store into the header section of the complex grid above the CountryName entry field.
- Double-click the label object and enter Country Name as the label text.
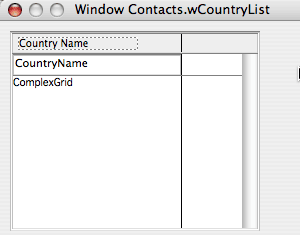

If the complex grid object is selected and you try to select an object inside the complex grid, clicking on the object inside the complex grid fails to select the object. To select an object inside of a complex grid (or any container object such as a tab pane, paged pane, group box, scroll box) hold down the Ctrl/Cmnd key and then click on the object. If you want to select a group of objects inside a container object hold down the Ctrl/Cmnd key and then drag a marquee around the objects.
Add a Save Button
We will now add a scrollbox container object and a pushbutton to the bottom of the window class.
- Click the Standard Fields icon in the F3 Component Store toolbar.
- Drag a Scroll Box object from the F3 Component Store into the lower area of the window class below the complex grid.
- With the scroll box object selected press F6 to open the F6 Property Manager and set the following properties.
General tab
$name - BottomContainer
$height - 50
Appearance tab
$edgefloat - kEFposnBottomToolBar
$fieldstyle - CtrlLabel
$effect - kBorderNone
$horzscroll - kfalse
$vertscroll - kFalse
- Drag a Push Button object from the F3 Component Store into the BottomContainer scrollbox object.
- With the push button object selected press F6 to open the F6 Property Manager and set the following properties.
General tab
$name - Save
$text - Save
$left - 325
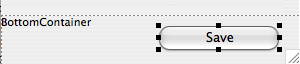
- Double-click the Save pushbutton object to get to the object's methods.
- Enter the following code in the $event method of the Save pushbutton object.
On evClick
If #SHIFT
Breakpoint
End If
Do iList.$dowork() Returns FlagOK
If not(FlagOK)
OK message [sys(85)] (Icon) {Flag false after $dowork}
Else
Do $cinst.$redraw()
End If

I like to include If #SHIFT, Breakpoint, End if just below the On evClick in the $event method of all pushbutton objects. I find this very handy for debugging code. If you want to step through the code, or look inside the window class instance you shift-click on any pushbutton and end up at the Breakpoint. From there you can continue stepping through the code or just look at the ivars and clear the method stack. You can add the If #SHIFT, Breakpoint, End if code to the pushbutton field object in the Component Store as follows:
- Right-click anywhere on the F3 Component Store window > select Show Component Library in Browser.
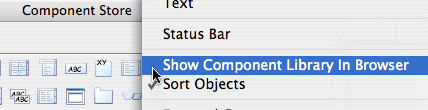
- F2 Browser > select Component Library library > double-click _Field Components
- Double-click the Push button object to get the object's methods.
- Add the following code to the $event method of the Push button field object.
On evClick
If #SHIFT
Breakpoint
End If
- Close the method editor window.
- While we are in the field components, lets modify the Scroll Box object default properties. Select the Scroll Box field object.
- F6 Property Manager > Appearance tab. Set the following properties:
Appearance tab
$fieldstyle - CtrlLabel
$effect - kBorderNone
$horzscroll - kfalse
$vertscroll - kFalse
- Close the _Field Components window.
- Right-click anywhere on the F3 Component Store window > select Show Component Library in Browser to uncheck the context menu item.
Test the Window
We are ready to test the wCountryList window class.
- Contacts menu > select Countries menu line. All going well an instance of the wCountryList window class will be opened, the $construct method will get all the records from the database, and the records will be listed in the complex grid.
- Change the name of one of the countries in the list by adding some extra letters to the end of the country name.
- Click the Save button.
- Close and reopen the Countries window. All going well the extra letters you added to the end of the country name will appear in the list.
- Remove the extra letters from the country name.
- Select the last country in the list and press the Tab key. The complex grid should automatically add a new line to the end of the grid.
- Enter a new country name, Germany.
- Click the Save button.
- Close and reopen the Countries window. All going well Germany will now be included in the list of countries.
Summary
In this section you have learned:
- How to create a window class, and add field components to the window class.
- How to modify components in the component store.
- How to get records and save changes from a window classs using an ivar list variable bound to a table class.