Tips_tutorials   >   Studio101   >   Startup Task
Startup Task
When the Contacts library is opened, Omnis Studio instantiates the Startup_Task and sends it a $construct message. (Omnis Studio call the $construct method)
Any code we want executed when the Contacts library is opened must be entered (or called from) the $construct method of the Startup_Task.
In this section we will add code to the startup task to open a session with the database and install a main menu.
Logon Session Object
We will use a task variable to instantiate a session object which will be used to open a session with the database. By using a task variable of the Startup_Task, the session will remain open as long as the Contacts library is open.
- F2 Browser > Contacts library > double-click Startup_Task.
- Variables list > Task tab > enter a new variable as follows:
Variable - dbsessionobj (Database Session Object)
Type - Object
- Subtype click the dropdown button > open the External Objects node > select OMSQLSESS
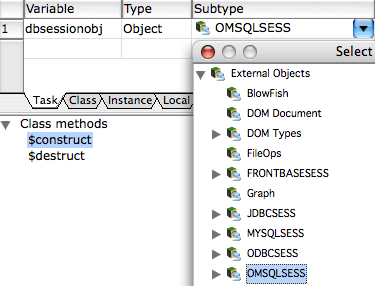
When the Startup_Task is instantiated, the dbsessionobj task variable will instantiate the OMSQLSESS external object. The object is used to open a session with the Omnis data file.
The next step is to add code which will open the session when the Contacts library is opened.
- Select the $construct method of the Startup_Task of the Contacts library.
- Enter the following code in the $construct method using the instructions immediately after this block of code. The instructions walk you through some helpful techniques for writing code in Omnis Studio.
Do FileOps.$splitpathname($clib.$pathname,Drive,FolderPath,FileName,Ext)
Calculate DataFilePath as con(Drive,FolderPath,'data',sys(9),'ContactsData.df1')
Do FileOps.$doesfileexist(DataFilePath) Returns FlagOK
If not(FlagOK)
OK message [sys(85)] (Icon) {Unable to find the data file.////File path: [DataFilePath]}
Else
Calculate HostName as DataFilePath
Calculate UserName as 'SYS'
Calculate Password as ''
Calculate SessionName as 'CONTACTS_01'
Do dbsessionobj.$logon(DataFilePath,UserName,Password,SessionName) Returns FlagOK
If not(FlagOK)
OK message [sys(85)] (Icon) {Unable to logon to the data file.////SessionName: [SessionName]////Host Name: [HostName]}
End If
End If
- Enter the comment line ; Calculate the path to the data file.
- Go to the next line, an empty method line, and type Do. This selects the Do command.
- Press the Tab key to move to the Calculation field.
- Press F9 to open the F9 Catalog > select Functions tab > select FileOps > select $splitpathname.
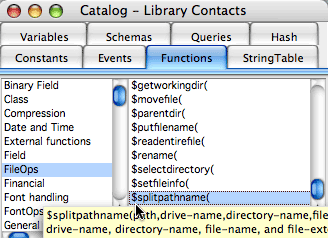
- Drag the $splitpathname method to the Calculation field of your Do command. Omnis Studio very kindly enters FileOps.$splitpathname with all of its parameters into the Calculation field for you.
- Change the output parameters to %% prefixed local variable names. e.g. Change drive-name to %%Drive.

Omnis Studio kindly creates %% prefixed character local variables on-the-fly for you.
If you were to use a single % character prefix Omnis Studio would create a floating point number local variable for you.
- Select the Local variables tab and remove the %% prefixes from the variable names.

It is not a good idea to leave % prefixed variables in your method code. If you use % prefixed variables and accidentally mistype the % prefixed variable Omnis Studio will create a new local variable on-the-fly. If this goes unnoticed you will have created a bug in your method which is difficult to detect.
- Continue entering the code. When you get to Do dbsessionobj.$logon(... use the Interface Manager as follows to help you enter the code.
- Select an empty method line and type Do. This selects the Do command.
- Press the Tab key to move to the Calculation field.
- Right-click on the dbsessionobj task variable in the Variables pane and select Interface Manager. This opens the Interface Manager window showing you all of the methods and properties available to you.
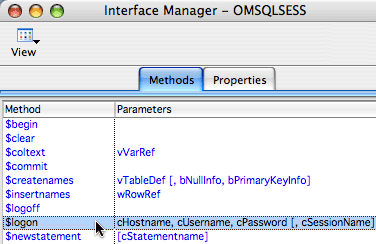
- Drag the $logon method to the Calculation field of your Do command.
Omnis Studio very kindly enters dbsessionobj.$logon and all the parameters in the Calculation field. All you need to do is replace the parameters with the actual input values. If you haven't yet created the input variables, you can add them on-the-fly by replacing the parameter name with a variable name that begins with %%. After Omnis Studio creates the local variables you can remove the %% prefixes if the Variables pane.
- Finish entering the $construct method code.
- Close and reopen the Contacts library startup task as follows.
- F2 Browser > Contacts library > right-click on the Startup_Task > select Close Task. This closes the startup task.
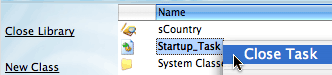
- Right-click again on the Startup_Task > select Open Task. This reopens the startup task. When the startup task is reopened Omnis Studio sends a $construct message to the task instance which causes our code in the $construct method to be run.
All going well the CONTACTS_01 session should appear as a child node of the SQL Browser node in the F2 Browser treelist.
Main Menu
We need to open some sort of a visual object to allow the user to interact with our application. The visual object could be a menu, toolbar, window class, or a combination of these classes.
To get things started we will create a menu class and install it from the $construct method of the Startup_Task.
- F2 Browser > select Contacts > click New Class > click Menu.
- Name the menu, mMainMenu.
- Double-click mMainMenu.
- Change the Omnis Studio menu class developer settings as follows:
- Reize the menu class window if it is too small.
- Right-click on the menu class window. If the Accept All Keystrokes context menu is checked, select it to uncheck it.
If Accept All Keystrokes is checked and you have a menu line selected in the menu window class when you press F6 to open the F6 Property Manager, instead of opening the Property Manager, Omnis Studio assigns F6 as the shortcut key combination for the selected menu line. That is why I am getting you to uncheck this property.
- Right-click on the menu class and select Save Window Setup. This saves the current menu class window size and the current Accept All Keystrokes setting to your Omnis Studio developer preferences for the next time you open a menu class window.
- Click the empty title of the menu window class and enter Contacts as the menu title.
- Press the Return key one or two times to create a new menu line.
- F6 Property Manager > General tab > set the following properties:
$name - Countries
$text - Countries...
- Double-click the Countries menu line in the menu class window to get to the menu line's methods.
- Add the following code to the $event method of the Countries menu line.

As per the StudioTips Naming Conventions local variable names that begin with a lower case r are item reference type variables. When you create the local variable rClass for the following code be sure to set the variable type to item reference.
Calculate ClassName as 'wCountryList'
Do $clib.$windows.$findname(ClassName) Returns rClass
If isnull(rClass)
OK message [sys(85)] (Icon) {Unable to find the window class '[ClassName]'.}
Else
Do rClass.$openonce('*') Returns rWin
If isnull(rWin)
OK message [sys(85)] (Icon) {Unable to open an instance of the window class [ClassName].}
End If
End If
Quit method rWin
- Close the method editor and click on the Countries menu line in the menu class window.
- Press the Return key twice to create two new menu lines.
- F6 Property Manager > General tab > set the following properties:
$name - ProgrammerTestMethod
$text - Programmer Test Method...
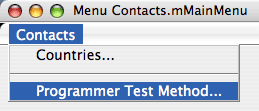
- Double-click the Progammer Test Method... menu line in the menu class window to get to the menu line's methods.
- Add the following code to the $event method of the ProgrammerTestMethod menu line.
Breakpoint
Quit method
The Programmer Test Method... menu line is a handy place to create and test code. We will be using the method in the next sections of this tutorial.
- To open an instance of the mMainMenu class during startup add the following code to the $construct method of the Startup_Task.
Calculate ClassName as 'mMainMenu'
Do $clib.$menus.$findname(ClassName) Returns rClass
If isnull(rClass)
OK message [sys(85)] (Icon) {Unable to find the menu class, [ClassName].}
Else
Do rClass.$open('ContactsMainMenu') Returns rMenu
If isnull(rMenu)
OK message [sys(85)] (Icon) {Unable to open the menu class, [ClassName].}
End If
End If
- Close and reopen the Startup_Task. All going well the Contacts main menu will be installed.
- Contacts menu > select Countries.... You should get an OK message stating that the wCountryList window class could not be found. This demonstrates why anticipating possible errors and including error messages in your code is helpful to your application development.